Tailwind CSS static navbar with shadow on scroll for Vue applications
Here's how to have a shadow on a static Tailwind navbar when the user scrolls the page for Vuejs applications
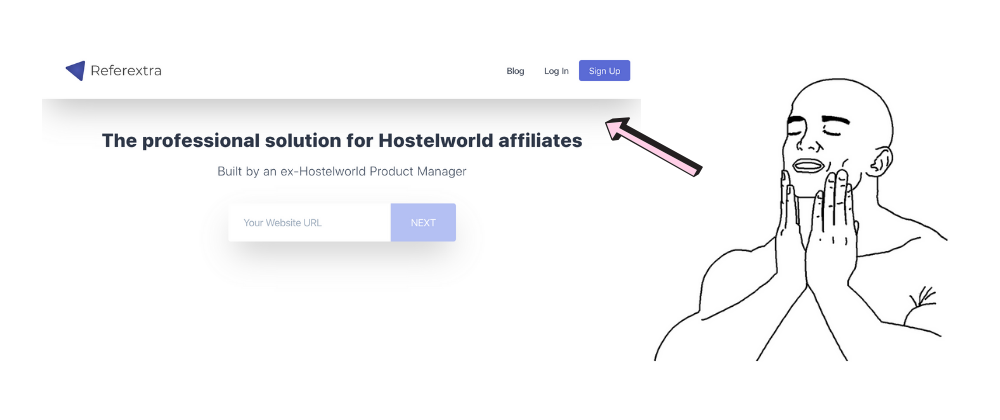
If you're not using Tailwind for your CSS needs - I highly recommend it. It's now a staple of my product building toolkit, and it just fits so well with Vue.js and Nuxt workflows that I can't imagine moving to something else.
One thing about Tailwind is it leaves the Javascript to you. This is so it's library-agnostic.
For most of my projects I want that smooth shadow under the navbar - here's the code I use to achieve it.
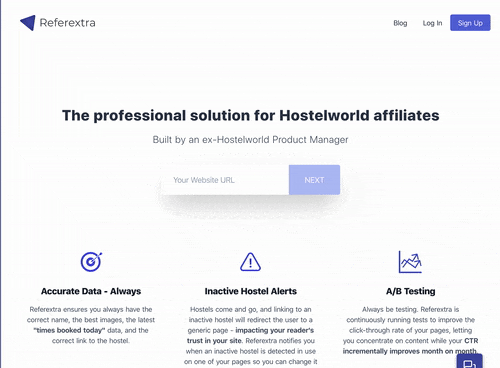
The HTML
<nav
:class="{ 'scrolled': !view.atTopOfPage }"
class="fixed flex w-full bg-white border-b items-center justify-between flex-wrap p-5 m-auto top-0 animated">
</nav>
Here, we're adding the .scrolled
class when the value in view.atTopOfPage
is false.
The CSS
nav {
z-index: 10
}
nav.scrolled {
@apply shadow-2xl;
border-bottom: 0px;
}
Apply the shadow to the navbar when it has the class scrolled
.
The Javascript
I have a navbar component that I use throughout the app, so this code would go there.
// in data, I like to store a view object with all
// the values I need for a component to manage
// it's 'view' state - ie loading,
// or in this case, if the user is at the top of the page or not
data () {
return {
view: {
atTopOfPage: true
}
}
},
// a beforeMount call to add a listener to the window
beforeMount () {
window.addEventListener('scroll', this.handleScroll);
},
methods: {
// the function to call when the user scrolls, added as a method
handleScroll(){
// when the user scrolls, check the pageYOffset
if(window.pageYOffset>0){
// user is scrolled
if(this.view.atTopOfPage) this.view.atTopOfPage = false
}else{
// user is at top of page
if(!this.view.atTopOfPage) this.view.atTopOfPage = true
}
}
}
The Result
Buttery smooth shadows on your navbar. Check it out in action on my product Referextra.com